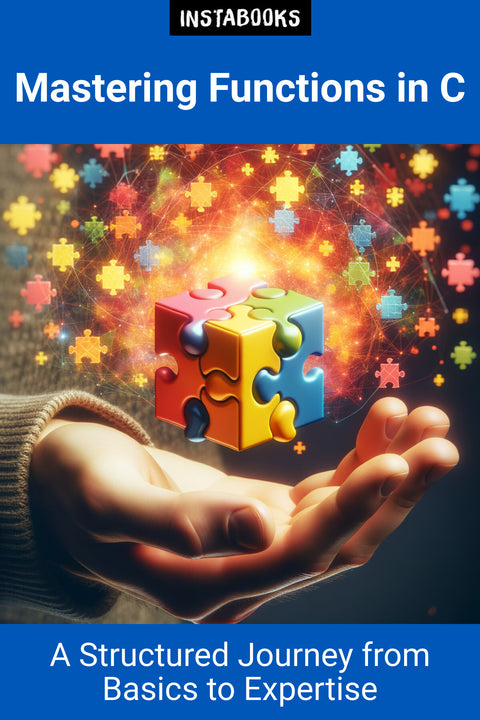
Mastering Functions in C
A Structured Journey from Basics to Expertise
AI Textbook - 100+ pages



Publish this book on Amazon KDP and other marketplaces
With Publish This Book, we will provide you with the necessary print and cover files to publish this book on Amazon KDP and other marketplaces. In addition, this book will be delisted from our website, our logo and name will be removed from the book, and you will be listed as the sole copyright holder.
$49.00
Dive into the intricate world of functions in C programming, an essential construct that enables modular, efficient, and reusable code. This book is tailored for programmers who aspire to deepen their understanding of functions, harnessing their full potential to create robust applications. The chapters meticulously guide you through various levels of function implementation, from simple to advanced concepts, ensuring you gain a well-rounded comprehension and skillset.
Begin your journey with foundational knowledge, exploring the purpose and basic syntax of functions in C. Each chapter progresses into more complex territories, unveiling the intricacies of function pointers, recursion, and libraries. For those who seek to excel, the book delves into optimization techniques and best practices to craft high-performance function code.
Not stopping at theory, this comprehensive guide provides practical examples and problem-solving exercises that animate the principles discussed. Your evolving expertise will empower you to tackle real-world programming challenges with confidence and finesse. As an educational resource for developers at all stages, 'Mastering Functions in C' stands as a beacon in the programming landscape, guiding you to excellence.
- Defining a Function: Syntax and Structure
- The Function’s Role in Modular Code
- Understanding Parameters and Return Types
2. Navigating Function Parameters
- Passing Values: Call by Value Method
- Working with References: Call by Reference
- Advanced Parameter Techniques
3. Returning from Functions
- Data Returns and Multiple Values
- Managing State with Return Codes
- Leveraging Pointers for Dynamic Returns
4. Storage Classes and Lifetimes
- Exploring Local, Global, and Static Variables
- Function Lifetimes and Their Implications
- The Impact of Storage Classes on Functions
5. Recursive Functions
- Understanding Recursion and Its Uses
- Designing Proper Base Cases
- Best Practices for Recursive Algorithms
6. The Power of Function Pointers
- Fundamentals of Function Pointers in C
- Practical Uses for Function Pointers
- Common Pitfalls and How to Avoid Them
7. Advanced Function Concepts
- Inline Functions and Macro Functions
- Variable-arity Functions: stdarg.h
- Nested Functions and Their Scope
8. Libraries and Functions
- Creating and Using Your Own Libraries
- Exploring Standard C Libraries
- Managing Dependencies
9. Error Handling in Functions
- Design Patterns for Error Reporting
- Exceptions and Assertions
- Building Robust Error Handling Mechanisms
10. Optimizing Functions for Performance
- Analyzing and Reducing Function Overhead
- Compiler Optimizations and Functions
- Micro-optimizations: When Every Cycle Counts
11. Debugging Function-Related Issues
- Common Function Bugs and How to Solve Them
- Using Debuggers Effectively
- Unit Testing and Functions
12. Best Practices and Design Patterns
- Writing Clean, Maintainable Function Code
- Functional Decomposition: A Strategy for Complexity
- Applying Design Patterns to Function Design
Table of Contents
1. The Essence of Functions- Defining a Function: Syntax and Structure
- The Function’s Role in Modular Code
- Understanding Parameters and Return Types
2. Navigating Function Parameters
- Passing Values: Call by Value Method
- Working with References: Call by Reference
- Advanced Parameter Techniques
3. Returning from Functions
- Data Returns and Multiple Values
- Managing State with Return Codes
- Leveraging Pointers for Dynamic Returns
4. Storage Classes and Lifetimes
- Exploring Local, Global, and Static Variables
- Function Lifetimes and Their Implications
- The Impact of Storage Classes on Functions
5. Recursive Functions
- Understanding Recursion and Its Uses
- Designing Proper Base Cases
- Best Practices for Recursive Algorithms
6. The Power of Function Pointers
- Fundamentals of Function Pointers in C
- Practical Uses for Function Pointers
- Common Pitfalls and How to Avoid Them
7. Advanced Function Concepts
- Inline Functions and Macro Functions
- Variable-arity Functions: stdarg.h
- Nested Functions and Their Scope
8. Libraries and Functions
- Creating and Using Your Own Libraries
- Exploring Standard C Libraries
- Managing Dependencies
9. Error Handling in Functions
- Design Patterns for Error Reporting
- Exceptions and Assertions
- Building Robust Error Handling Mechanisms
10. Optimizing Functions for Performance
- Analyzing and Reducing Function Overhead
- Compiler Optimizations and Functions
- Micro-optimizations: When Every Cycle Counts
11. Debugging Function-Related Issues
- Common Function Bugs and How to Solve Them
- Using Debuggers Effectively
- Unit Testing and Functions
12. Best Practices and Design Patterns
- Writing Clean, Maintainable Function Code
- Functional Decomposition: A Strategy for Complexity
- Applying Design Patterns to Function Design